react-query 개념 및 정리
react-query 개념 및 정리, react, react16, hook, useState, useRef, useMemo, useEffect, useReducer, useCallback, useQuery 동기적으로 실행
kyounghwan01.github.io
Overview
Overview React Query is often described as the missing data-fetching library for React, but in more technical terms, it makes fetching, caching, synchronizing and updating server state in your React applications a breeze. Motivation Out of the box, React a
react-query.tanstack.com
1. 설치하기
터미널을 실행시킨 후 아래 코드를 순서대로 입력한다.
- npx create-react-app my-app(프로젝트 이름, 대체 가능)
- cd my-app(프로젝트 이름)
- yarn add react-query
- yarn start
(React 버전임. Next.js에서는 다를 수 있음)
2. 기본 세팅하기
src/index.js 파일로 들어가서 아래와 같이 설정한다.
(왼쪽 - 설정 전 / 오른쪽 - 설정 후)
react-query를 적용했더니 local에서 실행했을 때 react-query 개발 도구가 생겼다. 신기😮
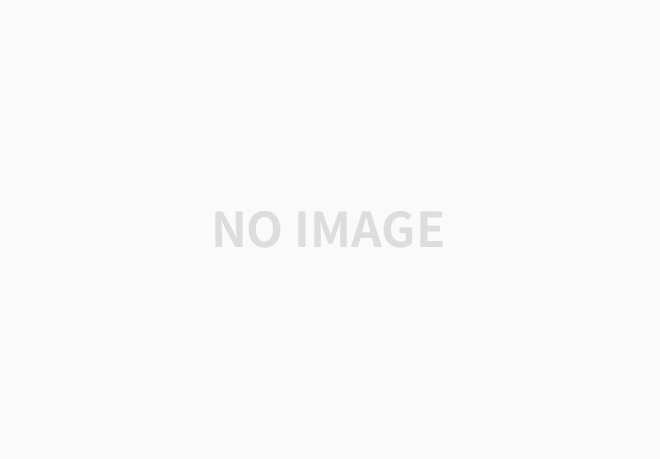
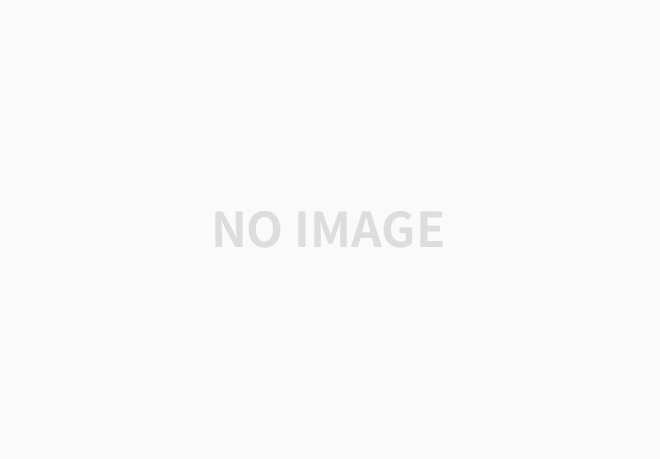
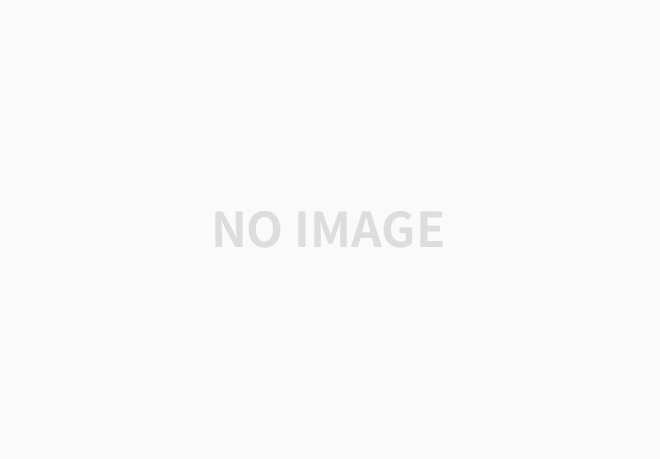

import React from "react";
import ReactDOM from "react-dom/client";
import "./index.css";
import App from "./App";
import reportWebVitals from "./reportWebVitals";
import { QueryClient, QueryClientProvider } from "react-query";
import { ReactQueryDevtools } from "react-query/devtools";
const queryClient = new QueryClient();
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<QueryClientProvider client={queryClient}>
{/* devtools */}
<ReactQueryDevtools initialIsOpen={true} />
<App />
</QueryClientProvider>
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
3. useQuery api 사용하여 fetch 해보기
: 데이터를 get 하기 위한 api입니다. post, update는 useMutation을 사용합니다.
첫 번째 파라미터로 unique key가 들어가고, 두 번째 파라미터로 비동기 함수(api 호출 함수)가 들어갑니다.
(두 번째 파라미터에는 promise가 들어가야 합니다.)
첫 번째 파라미터로 설정한 unique key는 다른 컴포넌트에서도 해당 키를 사용하면 호출할 수 있습니다.
unique key는 string과 array를 받습니다.
return 값은 api의 성공/실패 여부, api return 값을 포함한 객체입니다.
useQuery는 비동기로 작동합니다. 즉, 한 컴포넌트에 여러 개의 useQuery가 있다면 하나가 끝나고 다음 useQuery가 실행되는 것이 아닌 두 개의 useQuery가 동시에 실행됩니다.
여러 개의 비동기 query가 있는 구조라면 useQuery 보다는 useQueries를 사용하는 것이 더 좋습니다.
endbled를 사용하면 useQuery를 동기적으로 사용할 수 있습니다.
(예시)

import { useQuery } from "react-query";
const Example = () => {
const { isLoading, error, data } = useQuery("repoData", () =>
fetch("https://api.github.com/repos/tannerlinsley/react-query").then(
(res) => res.json()
)
);
if (isLoading) return "Loading...";
if (error) return "An error has occurred: " + error.message;
return (
<div>
<h1>{data.name}</h1>
<p>{data.description}</p>
<strong>👀 {data.subscribers_count}</strong>{" "}
<strong>✨ {data.stargazers_count}</strong>{" "}
<strong>🍴 {data.forks_count}</strong>
</div>
);
};
export default Example;
직접 실행해보니 devTools에서도 손쉽게 여러 가지 정보를 볼 수 있어서 좋았다. 공식문서나 다른 블로그들을 참고하면서 익숙해지다 보면 실제 프로젝트에서도 적용할 수 있을 것 같다!
'🧱 언어 모음집 > React Query' 카테고리의 다른 글
[React-query] Pagination에 Prefetching 사용하기 (0) | 2023.06.27 |
---|---|
[React-Query] react-query란? (2) | 2022.12.27 |
[React-Query] 개념 정리 (0) | 2022.06.15 |
댓글